Fred Vasquez
Documentation
Below, you will find more information about all the important systems/concepts used by the framework.
Action
An Action represents what an Actor is capable of doing on a particular turn. It can include everything from moving, to attacking, to equipping items, basically anything that you might want an actor to do. It provides functionality such as a cooldown timer, support for descriptions, etc. All actions have an energy cost, which is used by the turn-based system to know who goes when.
Actor
Actors represent the entities that the player will control and see. They all have an inventory, are capable of equipping items, have a stats system and are capable of leveling up after a certain amount of experience is gathered,
are able to move around the world, etc.
AI System
The AI system controls what Actors do when it is their turn to perform Actions. All Actors are created with an AI script attached, this script only has one function named "OnUpdate", which is called by the turn-based system when it identifies that the Actor should perform an Action. "OnUpdate" is in charge of populating the Actor's Action variable that will be performed that turn.
Client
The Client represents a system that listens to certain events that occur in Rog in order to deal with them gracefully. It is also the only script in Rog that uses Unity's update function, instead of a turn-based system.
Combat System
The Combat system is in charge of taking in 2 Actors that must battle it out and sending out combat events that happened, such as someone hitting another, someone dying, etc. It is also in charge of removing health from an Actor, dispensing experience points whenever an Actor kills the other, etc. It is very simplistic and makes very few assumptions on purpose, you should modify it any way you want.
Combat Events
These Events are called by the Combat system whenever 2 Actors are going at it. They include events such as OnLevelUp, OnKill, OnDeath, OnExperienceGained, etc. The combat system sends these events to the Actors and the Actors relay these events to all equipped items, in case you want items to do certain things during these events.
Item
Items represent objects that are used or equipped by Actors. They keep a reference of the Actor that owns the Item and can be either an equippable item, or a consumable item.
Map
A Map represents a 2D world where all Actors, Tiles, and Items live. It is basically a 2D array of MapNode objects. It offers many functions such as GetActorAt(x, y), etc. Maps are created by the dungeon and cave procedural generators.
MapNode
A MapNode represents a single point in a map and it stores everything that could possibly be at that particular point. It stores the geometry node (whether or not the point is walkable or not), a reference to an Actor (the actor that is currently placed at that position) as well as a list of items (items that may be dropped at that location).
Map Patch
A Map Patch is nothing more than a bunch of MapNodes stored as children of a gameObject. When a Map Patch is loaded, all the children of the gameObject are read and registered to the map at their appropriate locations. This is what allows the user to have both procedural maps mixed together with hand-crafted content, because when map patches are applied to the map, they overwrite whatever was at the position it was applied. Map Patches can only be created through the Map Editor, visit the FAQ to learn more.
Map Object
Any entity that can be placed on a map has a Map Object component attached to it. This component stores general information about the entity, such as whether or not it is solid, whether or not it emits light, whether or not it blocks light, and so on. Actors, Tiles, and Items are all map objects.
Turn-Based System
The turn-based system is what actually allows Actors to perform their actions. The list of registered actors is sorted based on each Actor's energy level, and the Actor is allowed to perform its action once its energy level passes a certain threshold.
Attribute
Before talking about Modular Statistics, we should talk about Attributes. These objects usually represent things such as health, defense, attack, etc.
They store 4 values:
- current value
- minimum value
- maximum value
- percentage value
Current, minimum, and maximum values are easy to understand, however, percentage works a little differently. It doesn't ever modify any of the other 3 values, instead, a temporal value which equals (current + percentage) is returned when retrieving an Attribute's current value.
Attributes also contain functionality that may prove useful to you, such as getting a ratio between current and max values, being able to modify all 4 values separately, etc.
Modular Statistics
This may be one of the hardest concepts to understand about Rog, but let me first explain why this system is used, as opposed
to a simpler version that represent stats such as health and attack with simple ints or floats.
Let's say you have an actor with a health value represented as a float, let's assume the value is 100. That actor now picks up an item, which increases that actor's health by a flat 5 points.
Health = 100 + 5 = 105.
Now let's say that the actor picks up another item, this time, the item increases that actor's health by 10%.
Health = 105 + 10% = 115.5
We're doing pretty good so far, but now, let's say that the actor wishes to remove the first item picked up (the one that gave a flat 5 increase.)
Health = 115.5 - 5 = 110.5
Now let's also remove the remaining item which gave a 10% increase.
Health = 110.5 - 10% = 99.45
Uh oh... we should be back to 100 health, instead, it has slightly decreased.
This problem is surprisingly complex to solve with a system that stores such values with simple ints and floats. Although they are easier to understand, they are very limiting and prone to errors.
Modular Statistics works very differently. Instead of modifying values all over the place (such as an item modifying the actor's health), each object stores its own values, called 'Statistics'. These Statistics objects are then all added together onto a temporal Statistics object, which is then what you see as their total. The values stored in the Statistics object are also not represented as ints or floats, they are represented with Attribute objects.
You request the actor's stats by calling the actor's 'GetTotalStatistics' function. Let's go over the same example as above with the new system.
Assume the actor has a Statistics object with a health value of 100 and a percentage of 0.
Assume item A has a Statistics object with a health value of 5 and a percentage of 0.
Assume item B has a Statistics object with a health value of 0 and a percentage of 10.
Health = HP of actor = (100) + (0%) = 100
Actor picks up item A.
Health = HP of actor + HP of item A = (100 + 5) + (0% + 0%) = 105
Actor picks up item B.
Health = HP of actor + HP of item A + HP of item B = (100 + 5 + 0) + (0% + 0% + 10%) = 105 + 10% = 115.5
Actor removes item A.
Health = HP of actor + HP of item B = (100 + 0) + (0% + 10%) = 110
Actor removes item B.
Health = HP of actor = (100) + (0%) = 100
As you can see, Statistics never modify each other's values, hence the name.
When you request an actor's stats, you simply get a temporal Statistics object that represents the stats of the actor plus the stats of all equipped items. This system not only solves the problem mentioned previously, but it is also quite easy to add more complex behaviour to with minimal work.
By default, an actor's 'GetTotalStatistics' call will return its stats plus the stats of all equipped items.
However, you can easily create more complex behaviour, for instance, let's say you want to add an action that passively gives the actor some health bonus. You could modify the actor's 'GetTotalStatistics' function to not only add together the stats of the items, but also the stats of all his actions!
And you don't have to stop there, if you want to have an item system like in Diablo, a system that adds affixes to items which modify their values, you could simply go to the item class, add an array of 'affix' objects, make sure they all have a Statistics object, and modify the item's 'GetTotalStatistics' by not only returning the items' stats, but by returning the item's stats plus the stats of all of its affixes. Simple!
Scripting System
Wouldn't it be nice if you could easily modify what happens to a particular actor or map object when a certain event occurs? With ROG 1.5 and above, now you can!
A map object now stores a 'Script Object', which contains a list of scripts that will run when particular events happen. A 'script' has virtual functions which will be called by ROG at appropriate times, such as when a turn ends, when an actor picks up an item, when an actor is damaged, etc. You can also easily add your very own events and decide when they are called too.
In order to create a new script you must do the following things:
-
Create a new .cs file in the Rog/ScriptSystem/Scripts_Custom folder (you can name it whatever you want).
-
Make sure the script extends 'ROG_ScriptCallback'.
-
Override any function you want in your newly created script.
-
Select any prefab with a map object component and add the name of your newly created script to the map object's script list.
NOTE: ROG 1.5 comes with YourScript.cs, take a look at that if you're not sure how to follow the points above.
A script object (which is what a map object stores) has the following important functions:
-
AddScript
-
RemoveScript
-
CallScript
NOTE: These functions are safe to call during run-time.


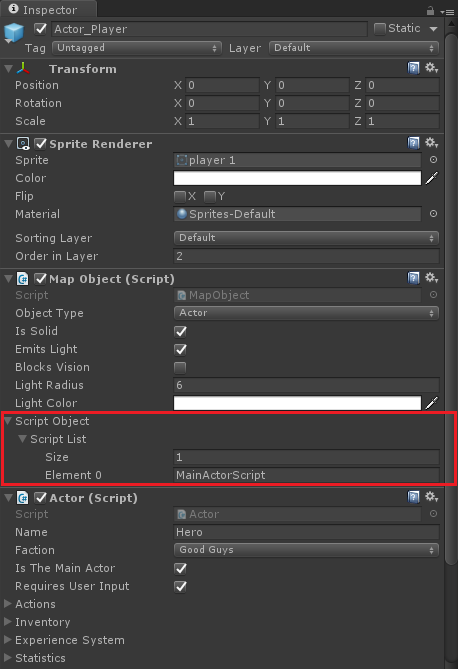